PlayerPrefs is a built-in utility in Unity 3D which provides the option to store certain types of data in the application. It makes the data sharing easier between scenes, PlayerPrefs can be created by setting a key/value pair, similar to dictionary or hashtable.
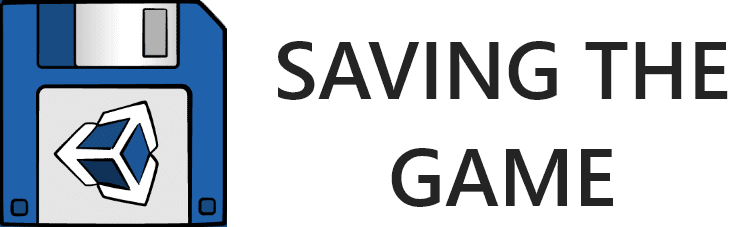
Today we’re going to see how to use the PlayerPrefs in Unity 3D for storing the game information like username, scores, and level information. PlayerPrefs in Unity 3D is limited to certain data types, so storing collections is not going to work in this.
List of Data Types and Functions Available in PlayerPrefs
Below listed items are the static methods in Unity PlayerPrefs, will see one by one.
SetFloat | Sets the Float value of the preference identified by a key. |
SetInt | Sets the Integer value of the preference identified by a key. |
SetString | Sets the String value of the preference identified by a key. |
HasKey | Returns true if the key exists in the preferences. |
GetFloat | Returns the Float corresponding to key in the preference file if it exists. |
GetInt | Returns the integer value corresponding to key in the preference file if it exists. |
GetString | Returns the value corresponding to key in the preference file if it exists. |
Save | Writes all modified preferences to disk. |
DeleteKey | Removes key and its corresponding value from the preferences. |
DeleteAll | Removes all keys and values from the preferences. |
Set methods are used to create/store the values into the preference. To set or create value in PlayerPrefs we need a key identifier for each value. Below are some example of setting the values
SetFloat
To set float value use the following method. PlayerPrefs.SetFloat("yourKey", value);
Here yourKey
is the key which is the identifier for your float value.
Ex: PlayerPrefs.SetFloat("playTime", 1.30f);
In this playTime
is the key and 1.30f
is the value which I’m going to save in the preference.
SetInt
Similarly, for integer value use the following method. PlayerPrefs.SetInt("yourKey", value);
Here yourKey
is the key which is the identifier for your integer value.
Ex: PlayerPrefs.SetInt("score", 4562);
In this playTime
is the key and 4562
is the value which I’m going to save in the preference.
SetString
And, for string value use the following method. PlayerPrefs.SetString("yourKey", value);
Here yourKey
is the key which is the identifier for your string/text value.
Ex: PlayerPrefs.SetString("playerName", "Tilak");
In this playTime
is the key and 4562
is the value which I’m going to save in the preference.
HasKey
HasKey
the method is used to check whether the given key is present in the PlayerPrefs when you’re going to retrieve the value. To avoid getting null
, it is recommended to check for the key. PlayerPrefs.HasKey("yourKey")
Here yourKey
is the key identifier which is holding your value.
This method will help you to verify the preference and manipulate the values.
Get methods are used to retrieve the values from the preference. To get value in PlayerPrefs we need to use the key identifier for each value. Below are examples of getting the values from the PlayerPrefs.
GetFloat
To get float value use the following method. PlayerPrefs.GetFloat("yourKey");
Here yourKey
is the key which is the identifier for the stored preference value..
Ex: PlayerPrefs.GetFloat("playTime");
In this playTime
is the key and it will return the values saved in the preference.
GetInt
For the integer value using the following method. PlayerPrefs.GetInt("yourKey");
Here yourKey
is the key which is the identifier for the stored preference value.
Ex: PlayerPrefs.GetInt("score");
In this score
is the key and it will return the values saved in the preference.
GetString
Same as to get String value use the following method. PlayerPrefs.GetString("yourKey");
Here yourKey
is the key which is the identifier for the stored preference value.
Ex: PlayerPrefs.GetString("playerName");
In this playerName
is the key and it will return the values saved in the preference.
Save
You can save all the modified preferences into the local disk by using this method. To save the preference use PlayerPrefs.Save();
This will store all preferences in the disk at this location.
On Windows standalone players, PlayerPrefs are stored in the registry under HKCU\Software[company name][product name] key
Web players, PlayerPrefs are stored in binary files under %APPDATA%\Unity\WebPlayerPrefs
on Windows.
Android – /data/data/<yourpackageName>/shared_prefs/<yourpackageName>.xml
Mac OS X PlayerPrefs are stored in ~/Library/Preferences
folder, in a file, named unity.[company name].[product name].plist
.
Where company and product names are the names set up in Project Settings.
DeleteKey
DeleteKey method is used to delete a key value from the preference, it will remove the preference value of the given key. Ex: PlayerPrefs.DeleteKey("playTime");
this will remove the key and its corresponding value from the preferences.
DeleteAll
DeleteAll method is used to clear all the key and values from the preference. As it can’t be undone use this method with caution. PlayerPrefs.DeleteAll();
this will remove all keys and values from the preferences.
Pros and Cons of PlayerPrefs in Unity
Even though using the PlayerPrefs to store and access is easier and fast, there are some points we have to keep in mind.
1. PlayerPrefs are not recommended to save sensitive data.
2. Stored data are not obfuscated or encrypted. Players game easily find the file and modify the information stored.
3. It is slower than the other methods.
4. WebGL build storage is limited to 1MB
5. And the file format for each platform is different, so multi-platform support is tough.
However, in my view, Serializing is a better and professional way to handle the data. Happy Coding!